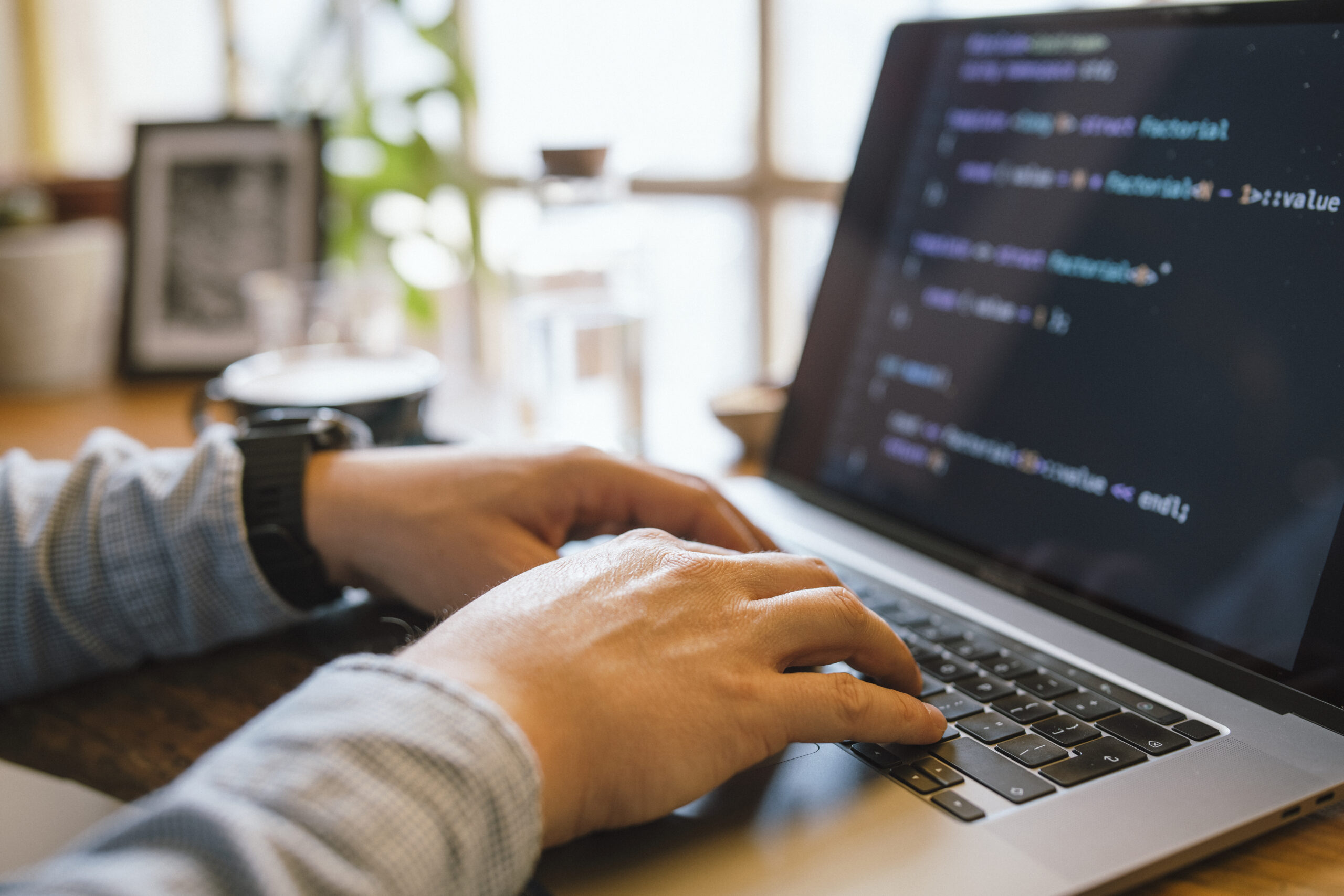
Debugging is one of the most essential — nevertheless normally overlooked — expertise within a developer’s toolkit. It isn't nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and Finding out to Assume methodically to unravel challenges competently. Irrespective of whether you are a newbie or even a seasoned developer, sharpening your debugging expertise can preserve hrs of disappointment and drastically help your efficiency. Here i will discuss various tactics that can help builders stage up their debugging video game by me, Gustavo Woltmann.
Grasp Your Resources
Among the fastest means builders can elevate their debugging competencies is by mastering the instruments they use every single day. Although creating code is one Element of development, recognizing tips on how to communicate with it successfully during execution is Similarly significant. Modern-day advancement environments come Geared up with effective debugging capabilities — but numerous builders only scratch the surface area of what these instruments can do.
Take, for example, an Integrated Development Ecosystem (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment let you established breakpoints, inspect the value of variables at runtime, action by way of code line by line, and in some cases modify code within the fly. When used the right way, they Enable you to notice particularly how your code behaves for the duration of execution, that is priceless for tracking down elusive bugs.
Browser developer equipment, for example Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, observe network requests, see serious-time efficiency metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can convert frustrating UI issues into manageable jobs.
For backend or system-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB present deep control above jogging processes and memory management. Finding out these tools can have a steeper Studying curve but pays off when debugging efficiency challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be relaxed with Edition Management devices like Git to be familiar with code history, locate the exact minute bugs were introduced, and isolate problematic adjustments.
Eventually, mastering your instruments usually means likely further than default options and shortcuts — it’s about establishing an personal familiarity with your progress ecosystem so that when issues occur, you’re not dropped in the dead of night. The greater you are aware of your applications, the greater time you are able to invest solving the actual problem rather than fumbling through the procedure.
Reproduce the issue
Probably the most crucial — and often missed — ways in productive debugging is reproducing the challenge. Right before leaping to the code or producing guesses, developers have to have to make a steady natural environment or scenario where by the bug reliably seems. Without having reproducibility, fixing a bug results in being a video game of prospect, generally resulting in wasted time and fragile code improvements.
Step one in reproducing a problem is accumulating just as much context as you can. Ask thoughts like: What actions led to The problem? Which atmosphere was it in — improvement, staging, or output? Are there any logs, screenshots, or mistake messages? The greater depth you have, the much easier it turns into to isolate the precise disorders below which the bug takes place.
After you’ve gathered adequate information and facts, try and recreate the challenge in your neighborhood surroundings. This may suggest inputting a similar info, simulating identical consumer interactions, or mimicking system states. If The problem seems intermittently, think about producing automatic exams that replicate the sting circumstances or point out transitions involved. These assessments don't just aid expose the situation but also reduce regressions Down the road.
Occasionally, The problem may very well be atmosphere-distinct — it'd come about only on certain working programs, browsers, or less than particular configurations. Making use of instruments like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the issue isn’t only a action — it’s a way of thinking. It needs endurance, observation, and also a methodical solution. But once you can regularly recreate the bug, you are previously midway to repairing it. That has a reproducible state of affairs, you can use your debugging tools much more successfully, check opportunity fixes properly, and connect extra Evidently with all your workforce or buyers. It turns an summary criticism right into a concrete obstacle — Which’s the place developers thrive.
Study and Comprehend the Mistake Messages
Error messages are frequently the most precious clues a developer has when one thing goes Improper. Instead of seeing them as aggravating interruptions, builders really should understand to deal with error messages as immediate communications with the technique. They normally inform you what exactly occurred, where it transpired, and often even why it occurred — if you know how to interpret them.
Begin by examining the concept very carefully and in whole. A lot of developers, specially when less than time strain, glance at the main line and quickly begin earning assumptions. But deeper in the error stack or logs may lie the genuine root result in. Don’t just duplicate and paste error messages into search engines like google — browse and recognize them 1st.
Break the mistake down into parts. Could it be a syntax mistake, a runtime exception, or possibly a logic mistake? Does it issue to a selected file and line quantity? What module or purpose induced it? These issues can manual your investigation and place you toward the dependable code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java generally follow predictable designs, and Discovering to recognize these can considerably speed up your debugging method.
Some faults are vague or generic, and in People conditions, it’s critical to look at the context by which the error transpired. Look at associated log entries, input values, and up to date improvements while in the codebase.
Don’t forget compiler or linter warnings possibly. These frequently precede greater difficulties and supply hints about potential bugs.
In the end, error messages are usually not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, supporting you pinpoint difficulties a lot quicker, reduce debugging time, and become a much more productive and self-confident developer.
Use Logging Correctly
Logging is One of the more impressive tools in a developer’s debugging toolkit. When utilized efficiently, it provides authentic-time insights into how an software behaves, serving to you have an understanding of what’s going on underneath the hood without having to pause execution or move in the code line by line.
A very good logging method begins with understanding what to log and at what level. Common logging levels include DEBUG, INFO, Alert, Mistake, and Lethal. Use DEBUG for specific diagnostic facts for the duration of growth, Data for basic occasions (like profitable get started-ups), Alert for prospective problems that don’t break the applying, ERROR for real problems, and Lethal in the event the process can’t proceed.
Steer clear of flooding your logs with too much or irrelevant facts. Excessive logging can obscure essential messages and decelerate your technique. Give attention to essential occasions, point out alterations, input/output values, and significant selection details with your code.
Format your log messages clearly and continually. Contain context, such as timestamps, ask for IDs, and function names, so it’s simpler to trace problems in dispersed units or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what problems are met, and what branches of logic are executed—all with no halting the program. They’re Specially valuable in output environments in which stepping as a result of code isn’t achievable.
Also, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with checking dashboards.
Eventually, clever logging is about stability and clarity. Which has a nicely-considered-out logging approach, you'll be able to lessen the time it takes to spot troubles, attain deeper visibility into your apps, and Increase the General maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not only a complex job—it's a sort of investigation. To correctly determine and resolve bugs, builders ought to solution the process like a detective resolving a secret. This mentality assists break down sophisticated troubles into workable sections and abide by clues logically to uncover the foundation result in.
Start off by collecting proof. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or general performance issues. Just like a detective surveys a crime scene, gather as much related info as you'll be able to without having jumping to conclusions. Use logs, check instances, and user reviews to piece collectively a clear image of what’s happening.
Subsequent, form hypotheses. Ask yourself: What can be producing this actions? Have any changes recently been built to your codebase? Has this challenge transpired prior to under identical situation? The purpose will be to slim down prospects and determine potential culprits.
Then, exam your theories systematically. Try and recreate the trouble inside a managed setting. In the event you suspect a particular function or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, inquire your code questions and Permit the outcomes direct you nearer to the reality.
Spend shut consideration to little aspects. Bugs typically hide during the minimum envisioned sites—just like a lacking semicolon, an off-by-one particular mistake, or possibly a race condition. Be extensive and affected person, resisting the urge to patch The difficulty with out thoroughly being familiar with it. Short-term fixes may perhaps conceal the actual difficulty, just for it to resurface later.
And lastly, maintain notes on That which you tried and acquired. Just as detectives log their investigations, documenting your debugging approach can save time for potential challenges and assist Some others understand your reasoning.
By contemplating just like a detective, builders can sharpen their analytical techniques, approach troubles methodically, and become more effective at uncovering concealed challenges in complicated programs.
Generate Tests
Creating exams is one of the simplest ways to increase your debugging techniques and overall improvement effectiveness. Assessments not only assist catch bugs early but additionally serve as a security Internet that gives you self-assurance when building changes on your codebase. A well-tested application is simpler to debug because it enables you to pinpoint particularly in which and when a difficulty happens.
Get started with device tests, which give attention to specific functions or modules. These little, isolated tests can speedily reveal whether a selected bit of logic is Performing as predicted. Each time a examination fails, you right away know the place to seem, substantially lowering the time spent debugging. Device assessments are Specially beneficial for catching regression bugs—problems that reappear after Beforehand currently being fastened.
Following, integrate integration checks and conclusion-to-conclude tests into your workflow. These help be sure that a variety of elements of your software get the job done collectively easily. They’re especially practical for catching bugs that arise in complicated systems with various elements or services interacting. If anything breaks, your tests can show you which Portion of the pipeline failed and less than what problems.
Writing assessments also forces you to Consider critically about your code. To check a function adequately, you'll need to be familiar with its inputs, anticipated outputs, and edge instances. This standard of comprehending Obviously prospects to higher code structure and less bugs.
When debugging a concern, writing a failing examination that reproduces the bug can be a strong initial step. As soon as the take a look at fails regularly, it is possible to deal with fixing the bug and look at your exam pass when The problem is solved. This approach ensures that the exact same bug doesn’t return Down the road.
In brief, producing tests turns debugging from a aggravating guessing video game right into a structured and predictable process—aiding you capture much more bugs, more rapidly plus more reliably.
Take Breaks
When debugging a tricky concern, it’s quick to become immersed in the issue—watching your display screen for hrs, hoping Alternative after Answer. But Just about the most underrated debugging equipment is actually stepping absent. Getting breaks helps you reset your mind, decrease disappointment, and sometimes see the issue from a new perspective.
When you're too close to the code for too long, cognitive fatigue sets in. You might start overlooking clear problems or misreading code which you wrote just hours earlier. In this point out, your Mind will become a lot less successful at dilemma-fixing. A short wander, a espresso crack, or maybe switching to a unique activity for 10–quarter-hour can refresh your aim. Quite a few developers report discovering the root of a problem when they've taken time and energy to disconnect, letting their subconscious work within the background.
Breaks also assistance protect against burnout, Specifically throughout longer debugging classes. Sitting in front of a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away allows you to return with renewed energy in addition to a clearer way of thinking. You would possibly out of the blue notice a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
If you’re caught, a good guideline would be to established a timer—debug actively for 45–sixty minutes, then have a 5–ten moment break. Use that point to move all over, stretch, or do anything unrelated to code. It may sense counterintuitive, Particularly less than tight deadlines, but it surely really brings about more rapidly and more practical debugging Eventually.
To put it briefly, using breaks is not really a sign of weak point—it’s a sensible method. It offers your Mind space to breathe, enhances your point of view, and helps you stay away from the tunnel vision That usually blocks your development. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Discover From Every single Bug
Each individual bug you experience is much more than simply A short lived setback—it's a chance to increase to be a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or simply a deep architectural issue, each one can educate you anything precious for those who make an effort to reflect and evaluate what went Mistaken.
Start out by inquiring you a handful of key concerns when the bug is solved: What brought about it? Why did it go unnoticed? Could it are caught before with improved tactics like device tests, code opinions, or logging? The responses generally expose blind places with your workflow or comprehension and allow you to Create more robust coding patterns going ahead.
Documenting bugs can be a fantastic behavior. Maintain a developer journal or preserve a log where you Take note down bugs you’ve encountered, the way you solved them, and Whatever you discovered. Over time, you’ll begin to see styles—recurring troubles or frequent errors—that you could proactively stay away from.
In group environments, sharing what you've acquired from the bug along with your peers is often Specially effective. Whether or not it’s via a Slack concept, a short generate-up, or a quick knowledge-sharing session, encouraging Other folks avoid the exact difficulty boosts crew efficiency and cultivates a more robust Understanding society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start off appreciating them as important portions of your advancement journey. After all, several of the best builders are not the ones who generate great code, but people who consistently discover from their faults.
In the end, Just about every bug you fix adds a completely new layer in your talent established. So up coming time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, much more capable developer thanks to it.
Conclusion
Bettering your debugging abilities normally takes time, observe, and patience — even so the payoff is large. It makes you a more productive, self-assured, and able developer. The next time you are knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become much better at Whatever click here you do.